MAUI has officially been released! With C# & XAML, we can now construct Native Mobile and Desktop applications. Yes, now we can create native Android, iOS, macOS, and Windows applications from a single code base. This is possible by using .NET’s new feature called Multi-platform App UI (MAUI). This is a cross-platform framework called .NET MAUI. MAUI, which stands for Multiple-Platform Application UI, allows us to create applications from a same code base for Android, iOS, Windows, and macOS. In addition, this implies that we can export apps to several platforms from a single project.
What is MAUI?
Multi-Platform App UI, or MAUI, is a free and open-source cross-platform framework. We can create native Android, iOS, macOS, Mac Catalyst, Tizen, and Windows applications using MAUI by using a single code base for all of these platforms.
The successor to Xamarin is the open-source .NET MAUI. Forms with UI controls rebuilt from the ground up for performance and extensibility, extending from mobile to desktop scenarios. You’ll see many similarities between .NET MAUI and Xamarin.Forms if you’ve previously used those tools to create cross-platform user interfaces. There are some differences, though. If necessary, you can add platform-specific source code and resources when developing multi-platform apps with.NET MAUI. The ability to implement as much of your app logic and UI layout in a single code base is one of the main goals of.NET MAUI.
The development of Xamarin.Forms from mobile to desktop applications led to MAUI. If you have experience with Xamarin.Forms, you will have no trouble understanding.NET MAUI.
The .NET MAUI is for such
- Developers who want to create cross-platform applications using C# and XAML from a single code base.
- Sharing the same UI layouts and designs across platforms, which reduces the time needed for design implementation.
- To use the same business logic, code, and testing across all platforms.
Install Visual Studio 2022 Preview
Only the Visual Studio 2022 Preview offers .NET MAUI right now. Obtain the Visual Studio 2022 Preview by clicking here.
Select .NET Multi-platform App UI Development Workload from the list below once it has been downloaded and installed.
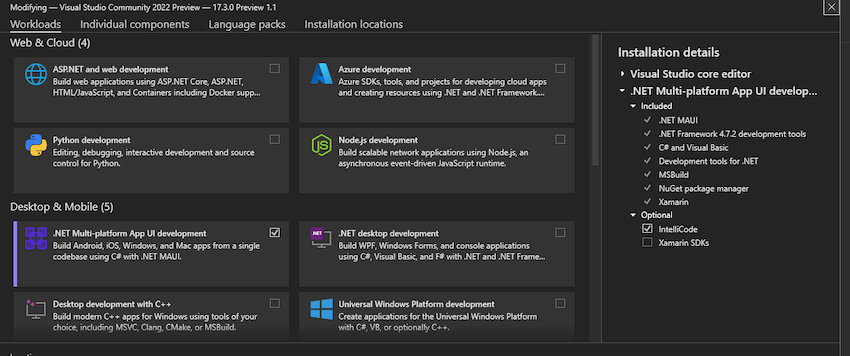
It’s best to restart the computer after a successful installation before starting Visual Studio 2022 Preview.
Making the first.NET MAUI application in the preview of Visual Studio 2022
At first, launch the Visual Studio 2022 Preview and Choose to create a new Project and search for MAUI.
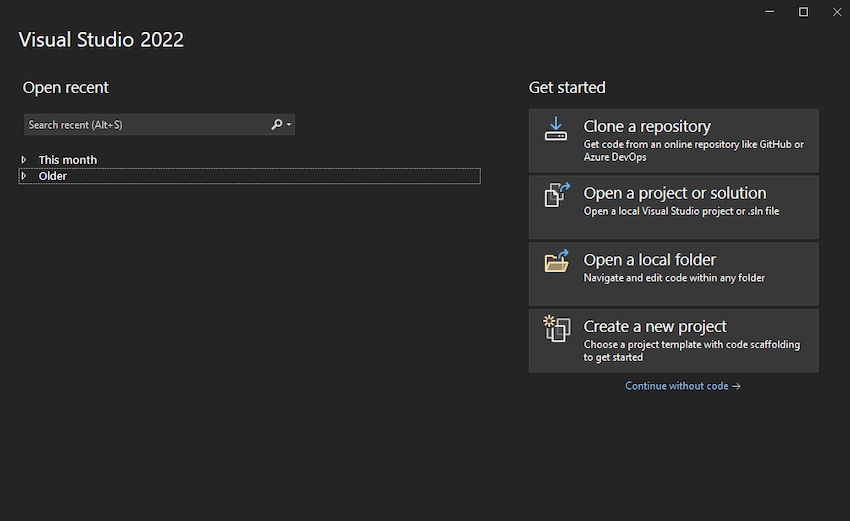
.NET MAUI has three categories, .NET MAUI App, .NET MAUI Blazor App, .NET MAUI Class Library.
In upcoming articles, we’ll examine each category in greater detail. The.NET MAUI App currently allows us to create applications from a single code base for Windows, Android, iOS, Mac Catalyst, MAUI, and Tizen.
.NET MAUI Blazor App – These applications are created using Blazor and can run on iOS, Android, Mac Catalyst, Tizen, and WinUI from a single source of code.
Applications can be run on Android, iOS, Mac Catalyst, macOS, Tizen, and Windows thanks to the .NET MAUI Class Library, which was used in their development.
Let’s create our first .NET MAUI App.
Choose to .NET MAUI App from the Project Template options and click Next.
Save it in the location by providing the project name.
.NET MAUI App uses XAML and is similar to WPF.
.NET MAUI Blazor
Now you may ask what is .NET Blazor?
It’s a feature of ASP.NET, the well-known web development framework, is called Blazor. It adds tools and libraries for creating web apps to the.NET developer platform.
Instead of using JavaScript, Blazor enables you to create interactive web user interfaces. Reusable web UI components are implemented in Blazor apps using C#, HTML, and CSS. Because C# is used for both client and server code, you can share code and libraries.
Using WebAssembly, Blazor can execute your client-side C# code right in the browser. You are able to reuse the code and libraries from the server-side components of your application because real.NET is running on WebAssembly.
You could also have Blazor execute your client logic on the server. Using SignalR, a real-time messaging framework, client UI events are returned to the server. The necessary UI changes are sent to the client and merged into the DOM once execution is finished.
In today’s discussion let’s use blazor to export multiple platforms
Using Blazor, we can easily export applications for Multiple Platforms.
Choose .NET MAUI Blazor from the Project Template while creating a new project and save it.
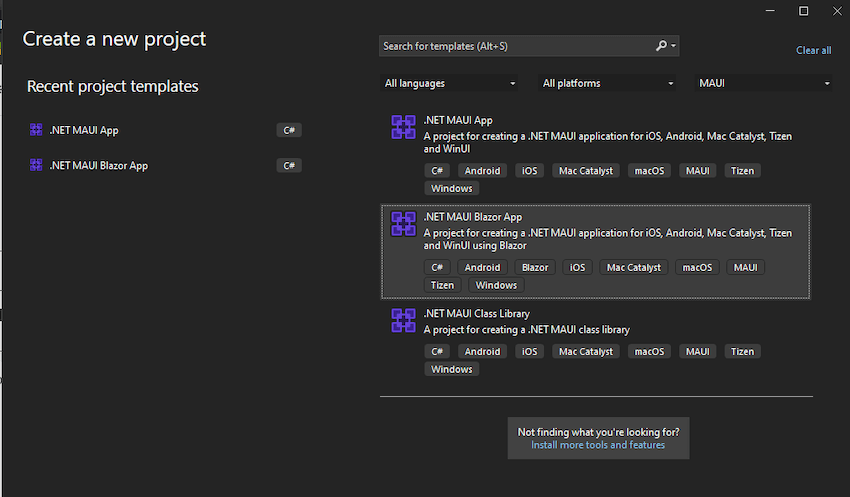
The default project folder structure is shown in the image below. It will be very simple for you to do this if you are already familiar with Blazor.
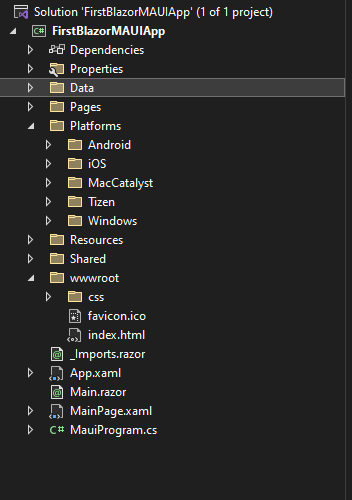
Similar options for exporting the application to various platforms are available for this project as they are for the.NET MAUI App. Let’s test the program on various platforms.
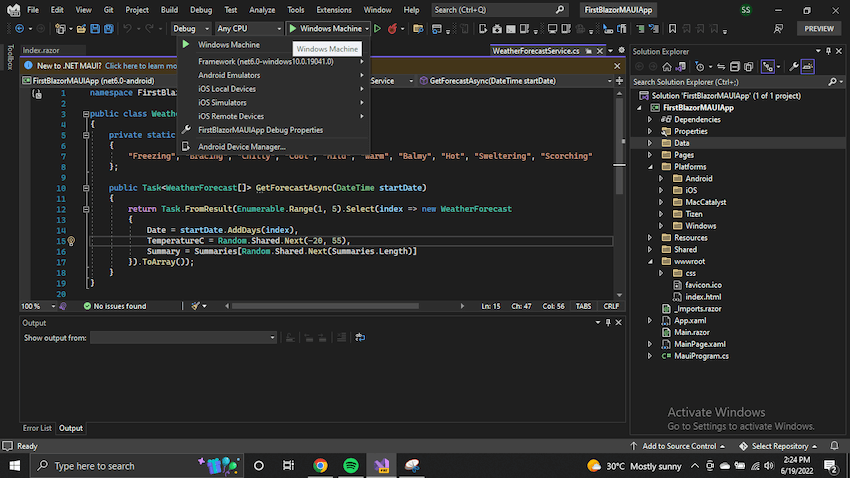
This project also has similar options to the .NET MAUI App to export the application to Mac, android or iOS platforms. But we will cover that in another article.
Conclusion
This article covered the creation of .NET MAUI applications and how to debug them across various platforms. The debugging for Windows devices, and procedure to create application in Mac, android and iOS devices have not been covered; they will be in subsequent articles later. But we hope that this article will help you to go through with everything related to MAUI if you are new here. Please feel free to share your feedback with us.