Jan 29
When showing a form that contains a TextBox, it’s common courtesy to focus the TextBox so that the user can begin typing immediately.
To focus a TextBox when a Windows Form first loads, simply set the TabIndex for the TextBox to zero (or the lowest TabIndex for any Control on the Form).
When a Form is displayed, it automatically focuses the Control with the lowest TabIndex. Note that if your TextBox is pre-initialized with some text, then the entire Text will be selected, as shown below:
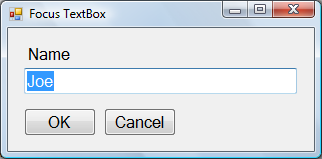
Read the rest of this entry »
Jan 15
Here is the code to read a text file from disk one line at a time into a string. This code ensures the file exists and properly closes the file if an exception occurs.
Read the rest of this entry »
Nov 19
This article discusses one of those programming topics that may be confusing at first but seems obvious once you know it.
As you know, C# enables you to overload the same method in a class with different arguments. But it’s also possible to overload a method with arguments that inherit from one another.
Read the rest of this entry »
Oct 22
Many objects in .NET are stored in a hierarchy. For example: controls, files and folders, and anything you would normally display in a tree view. There are many different algorithms for finding the root of a hierarchy. Here is one of them:
Read the rest of this entry »
Sep 03
It’s easy to determine if your C# application is 64-bit. Just check the Size property of IntPtr. If it’s 8, then your application is 64-bit. If it’s 4, then your application is 32-bit.
Here is a simple C# console program to demonstrate this:
Read the rest of this entry »
Sep 02
The DataGridView is a powerful grid control included in the .NET Framework. One function missing, however, is the ability to hide the current selection when the DataGridView control is not focused. What the DataGridView class needs is a HideSelection property, similar to the ListView and TextBox. But the .NET designers have not included this capability in the DataGridView class.
Read the rest of this entry »
Aug 30
The order of C# switch case statements in your code has no effect on performance.
Read the rest of this entry »
Aug 14
How do you sort a C# array in descending or reverse order? A simple way is to sort the array in ascending order, then reverse it:
Read the rest of this entry »
Jul 17
It’s not a trivial exercise to validate a file path on a Windows PC. There are a few special cases depending on the file system and operating subsystem:
Read the rest of this entry »
Jul 16
It’s easy to display an RTF file — that was embedded as a resource in a C# program — in a Windows Form RichTextControl.
Read the rest of this entry »