Jan 26
You may have noticed the first line of XML output generated by XmlWriter or XmlTextWriter shows that the encoding defaults to UTF-16:
<?xml version="1.0" encoding="utf-16"?>
This happens even if you explicitly set the Encoding property in the XmlWriterSettings to something different, such as UTF-8:
StringBuilder sb = new StringBuilder();
XmlWriterSettings settings = new XmlWriterSettings ();
settings.Encoding = System.Text.Encoding.UTF8;
XmlWriter writer = XmlWriter.Create (sb, settings);
The problem occurs because the StringWriter defaults to UTF-16. (It’s not clear from the example above, but the XmlWriter class uses a StringWriter to output the XML to the specified StringBuilder.)
Read the rest of this entry »
Jan 13
If you want to rename a file in C#, you’d expect there to be a File.Rename method, but instead you must use the System.IO.File.Move method.
You must also handle a special case when the new file name has the same letters but with difference case. For example, if you want to rename “test.doc” to “Test.doc”, the File.Move method will throw an exception. So you must rename it to a temp file, then rename it again with the desired case.
Here is the sample source code:
/// <summary>
/// Renames the specified file.
/// </summary>
/// <param name="oldPath">Full path of file to rename.</param>
/// <param name="newName">New file name.</param>
static public void RenameFile( string oldPath, string newName )
{
if (String.IsNullOrEmpty( oldPath ))
throw new ArgumentNullException( "oldPath" );
if (String.IsNullOrEmpty( newName ))
throw new ArgumentNullException( "newName" );
string oldName = Path.GetFileName( oldPath );
// if the file name is changed
if (!String.Equals( oldName, newName, StringComparison.CurrentCulture ))
{
string folder = Path.GetDirectoryName( oldPath );
string newPath = Path.Combine( folder, newName );
bool changeCase = String.Equals( oldName, newName, StringComparison.CurrentCultureIgnoreCase );
// if renamed file already exists and not just changing case
if (File.Exists( newPath ) && !changeCase)
{
throw new IOException( String.Format( "File already exists:n{0}", newPath ) );
}
else if (changeCase)
{
// Move fails when changing case, so need to perform two moves
string tempPath = Path.Combine( folder, Guid.NewGuid().ToString() );
Directory.Move( oldPath, tempPath );
Directory.Move( tempPath, newPath );
}
else
{
Directory.Move( oldPath, newPath );
}
}
}
May 01
To get the path of the current user’s temporary folder, call the GetTempPath method in the System.IO namespace:
string tempPath = System.IO.Path.GetTempPath();
On Windows Vista and 7, this method will return the following path:
C:UsersUserNameAppDataLocalTemp
Apr 21
It’s easy to read a file into a byte array. Just use the File.ReadAllBytes static method. This opens a binary file in read-only mode, reads the contents of the file into a byte array, and then closes the file.
string filePath = @"C:test.doc";
byte[] byteArray = File.ReadAllBytes( filePath );
Apr 12
The .NET FolderBrowserDialog class does not have an InitialDirectory property like the OpenFileDialog class. But fortunately, it’s quite easy to set an initial folder in the FolderBrowserDialog:
FolderBrowserDialog dialog = new FolderBrowserDialog();
dialog.RootFolder = Environment.SpecialFolder.Desktop;
dialog.SelectedPath = @"C:Program Files";
if (dialog.ShowDialog() == DialogResult.OK)
{
Console.WriteLine( dialog.SelectedPath );
}
Note that you can choose other RootFolder’s like MyComputer and MyDocuments. The SelectedPath must be a child of the RootFolder for this to work.
Given the sample code above, the dialog may look like this:
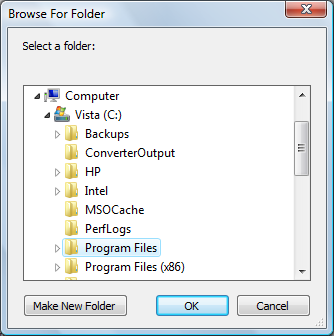
Feb 20
It’s easy to read a string one line at a time. Here is a console program that demonstrates how:
Read the rest of this entry »
Feb 19
It’s fairly easy to convert a C# String to a Stream and vice-versa.
Read the rest of this entry »
Jan 15
Here is the code to read a text file from disk one line at a time into a string. This code ensures the file exists and properly closes the file if an exception occurs.
Read the rest of this entry »
Jul 17
It’s not a trivial exercise to validate a file path on a Windows PC. There are a few special cases depending on the file system and operating subsystem:
Read the rest of this entry »
Jul 16
It’s easy to display an RTF file — that was embedded as a resource in a C# program — in a Windows Form RichTextControl.
Read the rest of this entry »