Oct 09
The standard Windows Forms ListBox control is not designed to display an icon with each item. You can modify the ListBox to handle the DrawItem event and manually draw the items and their associated icons, as shown here and here.
However, if you were hoping to use a standard off-the-shelf control with full icon support, your best bet is to use the ListView control.
Oct 09
To scroll a C# TextBox to the cursor/caret, it’s important that the TextBox is both visible and focused, then call the ScrollToCaret method:
textBox.Focus();
textBox.ScrollToCaret();
To scroll to the bottom/end of a TextBox, set the SelectionLength to 0 to remove any selection, then set SelectionStart to the end of the text in the TextBox:
textBox.SelectionLength = 0;
textBox.SelectionStart = textBox.Text.Length;
textBox.Focus();
textBox.ScrollToCaret();
Jun 10
This is one of those “D’oh!” moments. You’re creating your own UITypeEditor. You know the UITypeEditor class is located in the System.Drawing.Design namespace. So naturally you want to add to your Visual Studio project a reference to the System.Drawing.Design.dll, right? Wrong! When you compile your project, the following error may appear:
The type or namespace name ‘UITypeEditor’ could not be found (are you missing a using directive or an assembly reference?)
It turns out that UITypeEditor is actually defined in System.Drawing.dll, even though it’s located in the System.Drawing.Design namespace. See the disconnect? But you can easily solve this problem by adding to your Visual Studio project a reference to System.Drawing.dll.
Jun 08
When you create a Form with a border, Windows automatically draws a drop shadow around the form, as shown here:
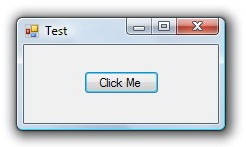
However, if you set the form’s FormBorderStyle property to None, Windows draws neither the form border nor the drop shadow, as shown here:
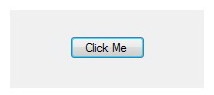
Read the rest of this entry »
May 01
To get the path of the current user’s temporary folder, call the GetTempPath method in the System.IO namespace:
string tempPath = System.IO.Path.GetTempPath();
On Windows Vista and 7, this method will return the following path:
C:UsersUserNameAppDataLocalTemp
Apr 21
It’s easy to convert a byte array to a string. For an ASCII string, use the Encoding.ASCII.GetString static method:
byte[] buffer = new byte[10];
// todo: populate the buffer with string data
string s = Encoding.ASCII.GetString( buffer );
Apr 21
It’s easy to convert a string to a byte array. For an ASCII string, use the Encoding.ASCII.GetBytes static method:
string s = "Test String";
byte[] byteArray = Encoding.ASCII.GetBytes( s );
Apr 21
It’s easy to read a file into a byte array. Just use the File.ReadAllBytes static method. This opens a binary file in read-only mode, reads the contents of the file into a byte array, and then closes the file.
string filePath = @"C:test.doc";
byte[] byteArray = File.ReadAllBytes( filePath );
Apr 12
The .NET FolderBrowserDialog class does not have an InitialDirectory property like the OpenFileDialog class. But fortunately, it’s quite easy to set an initial folder in the FolderBrowserDialog:
FolderBrowserDialog dialog = new FolderBrowserDialog();
dialog.RootFolder = Environment.SpecialFolder.Desktop;
dialog.SelectedPath = @"C:Program Files";
if (dialog.ShowDialog() == DialogResult.OK)
{
Console.WriteLine( dialog.SelectedPath );
}
Note that you can choose other RootFolder’s like MyComputer and MyDocuments. The SelectedPath must be a child of the RootFolder for this to work.
Given the sample code above, the dialog may look like this:
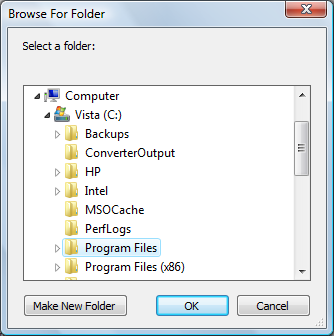
Mar 16
It’s easy to programmatically set the number of decimal places in a decimal, float or double that is converted to a string. Use the ToString method with the “N” argument summed with the number of decimal places:
float value = 3.1415926F;
int decimalPlaces = 3;
string text = value.ToString( "N" + decimalPlaces );
Note that ‘decimalPlaces’ must be greater than or equal to zero, otherwise an exception will be thrown.