Feb 05
Do you notice anything odd about the following list?
00000000-9b6d-4998-9dd7-6026894bdfba
11111111-9022-4400-bac2-8b66a9874443
22222222-a890-4dec-98bc-f41536b760bc
33333333-e361-4239-8d04-3f16f68ad9ce
44444444-d8c2-40ab-91bd-5a84511ed9d3
55555555-447a-4aa9-a51f-35c74a154156
66666666-193b-4ac3-bd92-860b6b49aedb
77777777-49de-4cc5-b9e6-2e5785dd47af
88888888-0d00-4672-933a-d68e240772be
99999999-7d9d-4d77-9e35-5e919db0f7d1
aaaaaaaa-76cd-4d6b-bae2-574e5b57c7ab
bbbbbbbb-6f9e-4d2d-ba11-64df5c7355fa
cccccccc-b897-4b15-9ab3-11b97836ce85
dddddddd-b417-48ad-8b5b-b762df75e03b
eeeeeeee-cc9c-4cb8-bae0-bbd4b10307fa
ffffffff-8d46-4a31-b297-2ac67dda3600
Read the rest of this entry »
Jan 13
If you want to rename a file in C#, you’d expect there to be a File.Rename method, but instead you must use the System.IO.File.Move method.
You must also handle a special case when the new file name has the same letters but with difference case. For example, if you want to rename “test.doc” to “Test.doc”, the File.Move method will throw an exception. So you must rename it to a temp file, then rename it again with the desired case.
Here is the sample source code:
/// <summary>
/// Renames the specified file.
/// </summary>
/// <param name="oldPath">Full path of file to rename.</param>
/// <param name="newName">New file name.</param>
static public void RenameFile( string oldPath, string newName )
{
if (String.IsNullOrEmpty( oldPath ))
throw new ArgumentNullException( "oldPath" );
if (String.IsNullOrEmpty( newName ))
throw new ArgumentNullException( "newName" );
string oldName = Path.GetFileName( oldPath );
// if the file name is changed
if (!String.Equals( oldName, newName, StringComparison.CurrentCulture ))
{
string folder = Path.GetDirectoryName( oldPath );
string newPath = Path.Combine( folder, newName );
bool changeCase = String.Equals( oldName, newName, StringComparison.CurrentCultureIgnoreCase );
// if renamed file already exists and not just changing case
if (File.Exists( newPath ) && !changeCase)
{
throw new IOException( String.Format( "File already exists:n{0}", newPath ) );
}
else if (changeCase)
{
// Move fails when changing case, so need to perform two moves
string tempPath = Path.Combine( folder, Guid.NewGuid().ToString() );
Directory.Move( oldPath, tempPath );
Directory.Move( tempPath, newPath );
}
else
{
Directory.Move( oldPath, newPath );
}
}
}
Oct 09
To scroll a C# TextBox to the cursor/caret, it’s important that the TextBox is both visible and focused, then call the ScrollToCaret method:
textBox.Focus();
textBox.ScrollToCaret();
To scroll to the bottom/end of a TextBox, set the SelectionLength to 0 to remove any selection, then set SelectionStart to the end of the text in the TextBox:
textBox.SelectionLength = 0;
textBox.SelectionStart = textBox.Text.Length;
textBox.Focus();
textBox.ScrollToCaret();
Jul 19
DeveloperFusion offers a free .NET code converter. Simply paste your C# or VB.NET code into this web-based tool, then select your target language: C#, VB.NET, Python or Ruby. Supports syntax up to .NET 3.5.
Code Converter
Jun 08
When you create a Form with a border, Windows automatically draws a drop shadow around the form, as shown here:
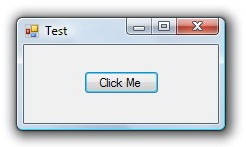
However, if you set the form’s FormBorderStyle property to None, Windows draws neither the form border nor the drop shadow, as shown here:
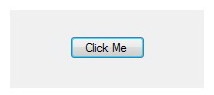
Read the rest of this entry »
Apr 21
It’s easy to convert a byte array to a string. For an ASCII string, use the Encoding.ASCII.GetString static method:
byte[] buffer = new byte[10];
// todo: populate the buffer with string data
string s = Encoding.ASCII.GetString( buffer );
Apr 21
It’s easy to convert a string to a byte array. For an ASCII string, use the Encoding.ASCII.GetBytes static method:
string s = "Test String";
byte[] byteArray = Encoding.ASCII.GetBytes( s );
Apr 21
It’s easy to read a file into a byte array. Just use the File.ReadAllBytes static method. This opens a binary file in read-only mode, reads the contents of the file into a byte array, and then closes the file.
string filePath = @"C:test.doc";
byte[] byteArray = File.ReadAllBytes( filePath );
Apr 12
Microsoft is releasing Visual Studio 2010, .NET Framework 4.0, and Silverlight 4 at the Visual Studio Developer Conference in Las Vegas. VS 2010 and .NET 4 are available today, and Silverlight 4 will be available to download later this week.
Read more at DevTopics >>
Jul 07
Microsoft is applying its Community Promise to the C# programming language and Common Language Infrastructure (CLI). This means that anyone can freely build, sell, distribute or use programs with C# and the CLI without signing a license agreement or otherwise communicating to Microsoft. This applies to all distribution models including open source and GPL. Under the Community Promise, Microsoft will not assert its Necessary Claims.
In other words, build all you want with C# and .NET, Microsoft won’t sue you for copyright or patent infringement.
Specifically, this announcement applies to the ECMA 334 (C#) and ECMA 335 (CLI) specifications.
“The Community Promise is an excellent vehicle and, in this situation, ensures the best balance of interoperability and flexibility for developers,” said Scott Guthrie, Corporate Vice President for the .NET Developer Platform.